Linear algebra for ML
2023. 11. 8. 20:47ㆍ학습/ML4ME[23-2]
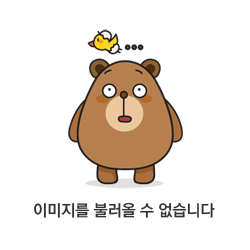
Quiz 1
from elice_utils import EliceUtils
import numpy as np
from numpy import array
from scipy.linalg import svd
elice_utils = EliceUtils()
def main():
M=array([[1,0,0,0,2],[0,0,3,0,0],[0,0,0,0,0],[0,4,0,0,0]])
# Task 1: Compute SVD
U, S, VT = svd(M)
# Task 2: Print out the shapes of these SVDs
print(U.shape, S.shape, VT.shape)
return U,S,VT
if __name__ == "__main__":
main()
Quiz 2
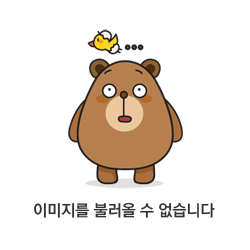
from elice_utils import EliceUtils
import numpy as np
elice_utils = EliceUtils()
def main():
# Task 1: Compute the pseudo_inv function without using any built-in pinv library
#TODO
A = np.array([[1, -3, 1],[-2, -1, -3], [-1, 1, 5], [0, 1, 1]])
# what should b yield?
#TODO
b = np.array([1, 5, -7, -3])
pinA = pseudo_inv(A)
# Task 2: Find the solution to the equation by solving the problem in Ax=b manner.
x = solve_linear(A,b)
# c) Rearrange the system of equations in the format By = 0 then find the optimal value of y
#TODO
B = np.array([[1, -3, 1],[-2, -1, -3], [-1, 1, 5], [0, 1, 1]])
optimal_y = solve_non_linear(B)
#elice_utils.send_image('elice.png')
#elice_utils.send_file('data/input.txt')
def pseudo_inv(A):
#TODO
A_transpose = np.transpose(A)
A_midinv = np.dot(A_transpose, A)
A_pinv = np.dot(np.linalg.inv(A_midinv), A_transpose)
return A_pinv
def solve_linear(A,b):
#TODO
x = np.dot(pseudo_inv(A), b)
return x
def solve_non_linear(B):
#TODO
U, S, VT = np.linalg.svd(B)
y = VT[-1] '''V의 마지막 행이 optimal solution'''
y /= y[-1] '''정규화'''
return y
if __name__ == "__main__":
main()
'학습 > ML4ME[23-2]' 카테고리의 다른 글
Parametric Density Estimation: Binary variable distribution (0) | 2023.11.12 |
---|---|
Probability (0) | 2023.11.09 |
Signal Processing (0) | 2023.11.08 |
Optimization Quiz (0) | 2023.11.08 |
Optimization (0) | 2023.11.07 |